Go Interfaces: Five Best-Practices for Enhanced Code Maintainability
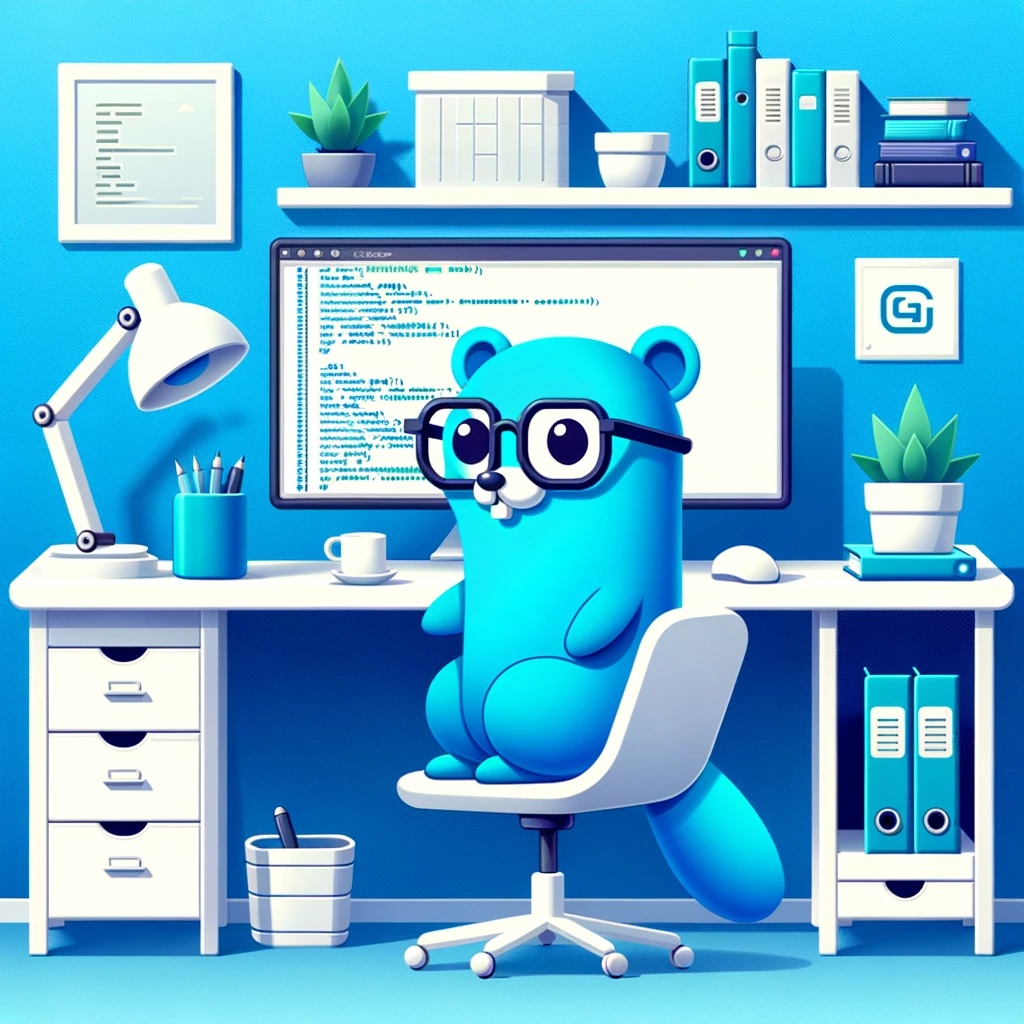
The Go maskot, a blue gopher, in front of a computer
In Go, an interface is a type that defines a method set. Its standout feature is implicit satisfaction: if a type possesses all the methods an interface requires, it automatically satisfies that interface. This is a key distinction from other languages, where explicit declaration is often necessary.
Implicit satisfaction offers greater flexibility and modularity, it also calls for careful attention to best practices. When developers adhere to these practices, they unlock numerous benefits.
First, it leads to cleaner and more organized code, as interfaces encourage the separation of concerns and a modular design. This modularity makes the code easier to test, maintain, and scale. Secondly, well-defined interfaces promote code reusability. They allow different parts of a program to communicate seamlessly, reducing redundancy and enhancing overall efficiency. Finally, following best practices in interface design enhances code readability and collaboration. It makes it easier for other developers to understand, use, and contribute to the codebase, which is crucial in team environments.
Best Practice 1: Define Interfaces Where They Are Used
Go interfaces generally belong in the package that uses values of the interface type, not the package that implements those values. 1
The first best practice can often seem unintuitive: to define interfaces where they are used, not where they are implemented. This approach has several advantages:
Encourages Loose Coupling
Defining interfaces in the consumer package leads to looser coupling between packages. This separation enhances flexibility and simplifies testing, as you can effortlessly create mock implementations of the interface for test scenarios. Example:
Consumer Package
type FileReader interface {
ReadFile(filePath string) ([]byte, error)
}
func ProcessFile(reader FileReader, filePath string) ([]byte, error) {
return reader.ReadFile(filePath)
}
Implementation package
type LocalFileReader struct {}
func (lfr LocalFileReader) ReadFile(filePath string) ([]byte, error) {
// Implementation for reading a file from local storage
}
Enhances Code Flexibility
When you define interfaces where they are used, they can be more specifically tailored to the needs of that particular package. This approach supports the development of more versatile and adaptable code structures.
Aligns with Dependency Inversion Principle
Aligning with the Dependency Inversion Principle of the SOLID principles, this approach ensures that both high-level and low-level modules depend on abstractions, not on concrete implementations.
classDiagram class FileProcessorModule { +FileReader reader +ProcessFile() } class FileReaderInterface { << interface >> +ReadFile(string) []byte, error } class LocalFileReader { +ReadFile(string) []byte, error } FileProcessorModule --> FileReaderInterface : uses LocalFileReader ..|> FileReaderInterface : implements
Best Practice 2: Keep Interfaces Small and Focused
In Go, smaller and more specific interfaces are usually better. This is often referred to as the “Interface Segregation Principle.” A well-designed Go interface:
- Contains only the methods that are necessary for the required functionality.
- Is easier to implement and understand.
- Allows for more reusable and interchangeable code components.
type FileSaver interface {
SaveFile(filePath string, data []byte) error
}
type FileRetriever interface {
RetrieveFile(filePath string) ([]byte, error)
}
Smaller interfaces are inherently easier to implement and understand. They reduce complexity, which in turn makes maintaining and updating the code more manageable. Focused interfaces lead to more reusable and interchangeable code components. They allow different parts of the application to interact more seamlessly, with each part having a clear and specific role.
Best Practice 3: Use Composition to Build More Complex Interfaces
Go’s design encourages the composition of interfaces, a powerful feature that allows developers to construct complex interfaces from simpler, more focused ones. Emphasizing composition over inheritance, this approach is fundamental in Go and plays a significant role in interface design.
Promotes Reusability and Clarity
Interface composition in Go enables the reusability of existing, smaller interfaces to build larger and more complex ones. This method ensures each interface remains focused and clear, while collectively they can represent more elaborate behaviours.
type FileSaver interface {
SaveFile(filePath string, data []byte) error
}
type FileRetriever interface {
RetrieveFile(filePath string) ([]byte, error)
}
type FileManager interface {
FileSaver
FileRetriever
}
Now, any type that implements both Read and Write methods implicitly satisfies the ReadWriter interface.
Simplifies Maintenance and Extensibility
By using smaller interfaces as building blocks, it’s easier to maintain and extend the code. Changes to one part of the system are less likely to impact others, making the codebase more robust and adaptable.
Aligns with Go’s Philosophy
This practice is in sync with Go’s overall philosophy of simplicity and efficiency. It allows developers to create flexible, powerful interfaces without introducing unnecessary complexity.
classDiagram class FileSaver { << interface >> +SaveFile(string, []byte) error } class FileRetriever { << interface >> +RetrieveFile(string) []byte, error } class FileManager { << interface >> } FileManager --> FileSaver : composes FileManager --> FileRetriever : composes
Best Practice 4: Understand the Zero Value of Interfaces
Understanding the zero value of interfaces in Go is fundamental, especially for those new to the language. This concept is critical in avoiding common bugs related to nil pointer dereferences.
In Go, an uninitialized interface holds a nil
value. However, there’s an important distinction: an interface that points to a concrete type with a nil
value is not the same as a nil
interface. This difference is key in preventing errors.
var fileManager FileManager // Uninitialized interface, nil
type CloudFileManager struct{}
func (cfm CloudFileManager) SaveFile(filePath string, data []byte) error {
// Method implementation
}
func (cfm CloudFileManager) RetrieveFile(filePath string) ([]byte, error) {
// Method implementation
}
var cfm *CloudFileManager
fileManager = cfm
if fileManager != nil {
fileManager.SaveFile("path/to/file", []byte("data"))
}
In this code, processor
is an interface assigned a nil concrete type (*StringProcessor
). It’s crucial to understand that processor
itself is not nil
, which can lead to unexpected runtime errors.
Always checking if an interface is nil
before invoking methods on it is an essential practice. This check helps prevent runtime errors and enhances the robustness of the code.
Best Practice 5: Design for Interface, Not Implementation
In Go, it’s a best practice to design your code with interfaces in mind, focusing on the behaviours you want to expose rather than on specific implementations. This practice encourages writing more flexible, scalable, and testable code.
Encourages Abstraction and Flexibility
By designing to an interface, you abstract away the details of the concrete implementation. This allows different parts of your code to communicate through well-defined contracts (interfaces), enabling you to change the underlying implementation without affecting the users of the interface.
type FileProcessor interface {
ProcessFile(filePath string, data []byte) ([]byte, error)
}
type EncryptionProcessor struct{}
func (ep EncryptionProcessor) ProcessFile(filePath string, data []byte) ([]byte, error) {
// Encrypt and process the file data
}
func processFileData(processor FileProcessor, filePath string, data []byte) ([]byte, error) {
return processor.ProcessFile(filePath, data)
}
var encryptor FileProcessor = EncryptionProcessor{}
processFileData(encryptor, "example/path", []byte("example data"))
In this example, the Storage
interface abstracts the storage operations, allowing FileStorage
or any other storage type to be used interchangeably as long as it satisfies the Storage
interface.
Improves Testability
Designing to interfaces makes testing easier. You can create mock implementations of the interface for testing, without relying on the concrete implementation, leading to more modular and isolated tests.